Arrays
An Array is an ordered list of values. Each value is called an element, and each element has a numeric position in the Array, known as its index.
An element can be any type of data, eg. a String, a Boolean, a Number, or even an Object or another Array. An element can also be a dynamic value, eg. system.userInput
. Each element can have a different type.
An Array is contained within two square brackets: []
, and its elements are separated by comas. For example, this Array: ["one", 1]
contains two elements: the Atring "one"
and the Number 1
.
Arrays are commonly used to store, process and send data. In Automate, you may meet them while using NLU API, Knowledge Base, integrations with external databases etc.
In Automate, you can create, access, and modify Arrays using Expression language (AEL) or JavaScript (JS).
An Array without any element that is an Object or an Array is called a one-dimensional Array.
Below, you will find a list of all operations and methods that can be performed on Arrays.
Using multiple methods at once
You can perform complex operations on Arrays (and other data types) by joining methods together.
Each time you use any of the methods listed below, you get a result instantly. Then, you can use another method in the same line to be performed on this result, and so on...
For example, to skip the first three elements of an Array and check the length of this new, shorter Array, you could write such expression:
[1, 3, 5, 7, 9].skip(3).length()
Result:2
Full list of Array methods
The following table contains a list of 3 essential Array operations that are further explained below:
Essentials | Description |
---|---|
Create an Array | Initialize an Array using literal constructor, eg. ["one", "two"] . Learn more |
Access an element by index | Access a specific element using its index [i] . Learn more |
For each loop | Iterate through an Array's elements to join and print its elements. Learn more |
The following table contains all methods used to process and modify Arrays in Expression language. Click on a link for more information and examples:
Methods | Description | Argument | Result |
---|---|---|---|
add() | Adds a new element to an existing Array. | any* | Array |
concat() | Creates a new Array by joining two Arrays into one. | Array | Array |
contains() | Checks if an Array contains given element. | any* | boolean |
exists() | Checks if an Array contains an element that satisfies given conditions. | function | boolean |
filter() | Creates a new Array with elements that satisfy given conditions. | function | Array |
find() | Returns the first element that satisfies given conditions. | function | various* |
first() | Creates a new Array from the first n elements of an Array. | number | Array |
join() | Creates a String by joining all elements of an Array. | String | String |
last() | Creates a new Array from the last n elements of an Array. | number | Array |
length() | Returns a number of elements of an Array. | - | number |
map() | Creates a new Array by applying a specified function to every element of an Array. | function | Array |
skip() | Creates a new Array by excluding the first n elements from an Array. | number | Array |
sort() | Sorts an Array of elements with compatible types in an ascending order. | - | Array |
sortDesc() | Sorts an Array of elements with compatible types in an descending order. | - | Array |
sortBy() | Sorts an Array of Objects by a specified property in an ascending order. | function | Array |
sortByDesc() | Sorts an Array of Objects by a specified property in an descending order. | function | Array |
sum() | Returns a sum of the numbers stored in an Array. | Array of numbers | number |
unique() | Creates a new Array containing unique values. | - | Array |
_any
- any data type, eg. a String, a boolean, or an Object. Learn more about data types.
_various
- any data type. It is identical to the original element.
Create (initialize) an Array
To create an Array (e.g. when setting variable in memory), simply input wished Array using square brackets and comas. In JS, it is called 'literal constructor'.
Examples:
// Create an Array with 4 elements - 2 numbers and 2 Strings:
[1, 2, "foo", "bar"]
// Create an empty Array:
[]
// Tip: create an empty Array so you can later add elements to it with add()
// Create an Array with two elements that are variables stored in Memory:
[memory.firstStep, memory.secondStep]
// Create an Array with two elements that are Objects:
[
{
firstName: "Michael",
lastName: "Scott"
},
{
firstName: "James",
lastName: "Halpert",
age: 34
}
]
In Automate, you can initialize an Array as a part of AEL expression, in any type of input field. Usually, you will see Arrays that were created to be stored in Memory or Knowledge Base:
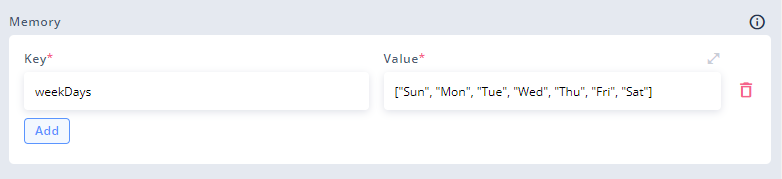
Create and store an Array with shorten versions of weekdays in Memory
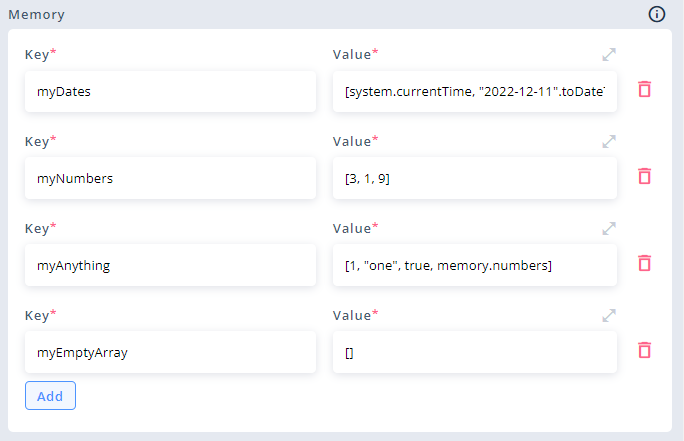
Other examples of how an Array can be created
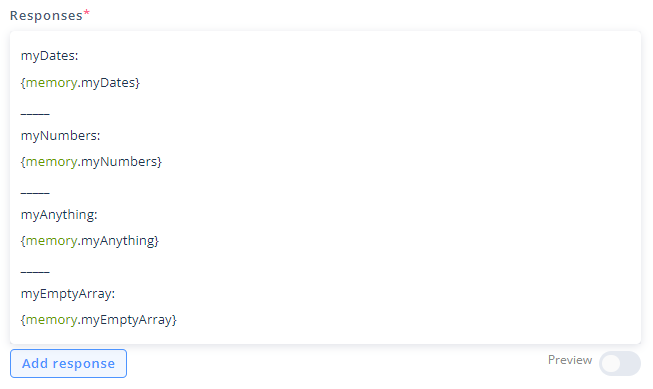
You can print the whole array in a Response.
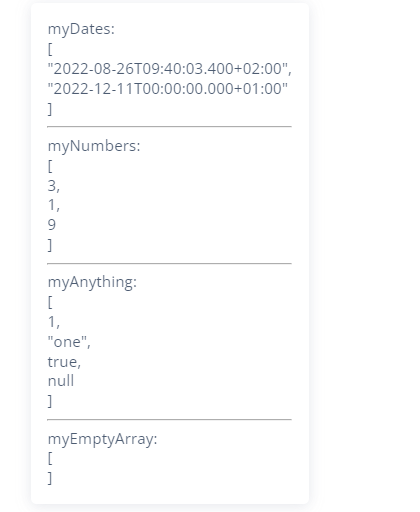
Example of how the output will look
Access an element by index
Index is a number stating position of the element in an Array.
To access an element by its index, add it directly after an Array (or a variable storing an Array - see Example 2), wrapped in square brackets.
[0] is the index of 1st element
Beware that in AEL and JS, the first element of an Array has index 0.
That's why, to access the first element, use[0]
, to access the second one, use[1]
, etc.
Calling an index outside of Array's length
If you try to call an index that is too high, an error will occur.
For example, if an Array's length equals 3 (it has three elements), then you can call up to three indexes:
[0]
,[1]
, or[2]
. Calling[3]
or anything higher on this Array will result in an error.To make sure that you won't call an index that is too high, check it's length().
Examples:
// Access the fourth element of an Array:
[1, 2, "foo", "bar"][3]
// Result:
"bar"
// Access the first element of an Array stored in memory.someArray:
memory.someArray[0]
// For memory.someArray = ["English", "Polish", "Italian"]
// the result is:
"English"
// Access an element of an Array with an index stored in a variable:
[1, 2, "foo", "bar"][memory.someIndex]
// For memory.someIndex = 2
// the result is :
"foo"
// Access an element of an Array which itself is an element of a nested (outer) Array:
[1, 2, ["Jim", "Pam"], "Michael"][2][1]
// Result:
"Pam"
// Explanation:
// [2] is accessing the third element of a nested Array returning ["Jim", "Pam"],
// and then [1] is accessing the second element of this inner Array, returning "Pam".
For each loop
Foreach is used to iterate through an Array, transform it's elements, combine them together and print as a String (with new line character as a delimiter).
-
Start the epression with
foreach
keyword. It is followed by the variable's name of your choosing and the keywordin
. You will use this selected name to access the current element in an Array. For example, the start of the expression can look like that:foreach product in
orforeach element in
. -
Next, input an Array that you want to iterate through (or a variable storing an Array, eg.
nlu.variablesList
). -
Finally, compose an interpolated String in a new line and inside double quotation marks. Access and modify the current element of an Array with the name you selected by interpolating (throwing in) an expression inside curly brackets.
Examples:
// Iterate through a one-dimensional Array of numbers and multiply them by 2:
foreach element in [1, 2, 3]
"{element * 2}"
// Result:
2
4
6
// Iterate through multi-dimensional Array (an Array of Objects)
// that stores loan products in memory.products to print a readable message:
// Expression:
foreach product in memory.products
"{product.type} no. {product.num}, remaining debt: $ {product.amount}"
// For memory.products:
[
{
type: "Student loan", amount: 10020, num: "SL324"
},
{
type: "Quick loan", amount: 2450, num: "QL222"
}
]
// Result:
Student loan no. SL324, remainig debt: $ 10020
Quick loan no. QL222, remainig debt: $ 2450
If you don't want to print all elements, you can use first()
, last()
or skip()
functions on an Array, for example:
// Iterate through a one-dimensional Array of numbers and multiply them by 2
foreach element in [1, 2, 3].first(2)
"{element * 2}"
// Result:
2
4
add()
Adds one or more elements at the end of an Array.
Element can be any type of data, including Array and Object. add()
without any argument throws an error.
- Syntax:
Array.add(new_elements)
- Result:
Array
Examples:
// Add a new element to an Array:
["Meredith", "Kevin"].add("Todd")
// Result:
["Meredith", "Kevin", "Todd"]
// Add multiple elements to an Array
["Meredith", "Kevin"].add("Todd", "Ryan")
// Result:
["Meredith", "Kevin", "Todd", "Ryan"]
// The variable memory.accountants stores an Array which is a list of accountants. The new one named Kevin was hired,
// so we want to add him to our Array.
// Initial value stored in memory.accountants:
["Oscar", "Angela"]
// Use add() on memory.accountants:
memory.accountants.add("Kevin")
// New value stored in memory.accountants:
["Oscar", "Angela", "Kevin"]
// You can add any type of data as a new element, eg. another Array:
["some String", 22, 24.4].add(["some other String", true, 14])
// Result:
["some String", 22, 24.4, ["some other String", true, 14]]
concat()
Creates a new Array by joining two Arrays into one.
- Syntax:
Array.concat(joined_Array)
- Result:
Array
Examples:
// Concatenate two Arrays:
[1, 2, 3].concat(["five", "seven"])
// Result:
[1, 2, 3, "five", "seven"]
// Each of the concatenated Arrays can be stored as a variable in Memory, Knowledge Base,
// or be taken from API response (in this case, NLU API):
memory.arr1.concat(nlu.variablesList)
// For memory.arr1 equal to:
[
"some String",
2
]
// and nlu.variablesList equal to:
[
{
name: "bank_transfer",
score: 0.9
},
{
name: "help",
score: 0.2
}
]
// Result:
[
"some String",
2,
{
"name": "bank_transfer",
"score": 0.9
},
{
"name": "help",
"score": 0.2
}
]
contains()
Checks if an argument is identical to at least one of the Array's elements.
Just like the Array's elements, an argument of contains()
can be of any type (String, boolean, number, Object etc.).
To return true
, the argument and the element of an Array have to be of the same type.
For example, [1, 2].contains("1")
will return false
.
- Syntax:
Array.contains(element_to_check)
- Result:
boolean
(true
orfalse
) - Similar methods: exists(), filter(), find()
Examples:
// Check if an Array contains the number 4:
[2, 3, 4, 5].contains(4)
// The result:
true
// For:
memory.arr1 = ["4", "5", "11"]
memory.arr2 = [4, 5, 11]
nlu.variables.numeral.value = 4
// Check if the first Array (memory.arr1) contains the number recognized by NLU:
memory.arr1.contains(nlu.variables.numeral.value)
// Result:
false
// Check if the second Array (memory.arr2) contains the number recognized by NLU:
memory.arr2.contains(nlu.variables.numeral.value)
// Result:
true
exists()
Checks if an Array contains an element that satisfies given conditions.
Unlike with contains()
, here you can use arrow function to check any condition, not just the identicalness of an argument and Array's elements.
This method is useful to check Arrays of Objects. It lets you check if the condition is fulfiled for a specific property of Objects that are elements of an Array (see example 2).
- Syntax:
Array.exists(function)
- Result:
boolean
(true
orfalse
) - Similar methods: contains(), filter(), find()
Examples:
// Check if an Array contains an element that is larger than 5 (an element has to be a number, too):
[1, 2, 6].exists(el => el > 5)
// Result:
true
// For memory.arr1:
[
{
Answer: 1,
QuestionId: 2
},
{
Answer: 3,
QuestionId: 5
}
]
// Check if an Array stored in memory.arr1 contains an Object that has a property Answer
// which value is equal to 1:
memory.arr1.exists(el => el.Answer = 1)
// Result:
true
// Check if there is an element that after using replace() on it will return "I'm 14":
["5", "10", "14"].exists(el => "I'm ??".replace("??", el) = "I'm 14")
// Result:
true
filter()
Creates an Array from the elements of an initial Array that satisfy given conditions.
This method uses arrow functions that let you create both simple and complex conditions. You can also add a few conditions using AND and OR operators.
- Syntax:
Array.filter(function)
- Result:
Array
- Similar methods: contains(), exists(), find()
Examples:
// Filter an Array so that the new Array has only elements larger than 3:
[1, 2, 4, 6].filter(el => el > 3)
// Result:
[4, 6]
find()
Returns the first element of an Array that satisfies given conditions.
This method uses arrow functions that let you create both simple and complex conditions. You can also add a few conditions using AND and OR operators.
If an Array doesn't contain any element that satisfies given conditions, the result will be null
.
- Syntax:
Array.find(function)
- Result:
element
- Similar methods: contains(), exists(), filter()
Examples:
[10, 20, 30].find(el => el >= 20)
// Result:
20
// This Array contains two Objects. Find the first element (Object) that has a property Answer equal to 5:
[{Answer: 1, QuestionId:"asd"},{Answer:5, QuestionId:"casd"}].find(el => el.Answer = 5)
// Result:
{Answer:5, QuestionId:"casd"}
first()
Creates a new Array that consists of the first n elements of an initial Array.
If an argument (number of elements to get) is equal to or higher than the length of an initial Array (number of all elements), then the new Array will be identical to the initial one.
Examples:
[1, 2, "foo", "bar"].first(2)
// Result:
[1,2]
join()
Creates a String from all of the elements of an Array.
The String is composed from the elements ordered accordingly to the initial Array. Each element is separated by a delimiter defined in an argument (delimiter_String).
Elements of the type different from String will be parsed to String, so you can use this method with any Array.
- Syntax:
Array.join(delimiter_String)
- Result:
String
- See also: For each loop
Examples:
[1, 2, "foo", "bar"].join(",")
1, 2, "foo", "bar"
last()
Creates a new Array that consists of the last n elements of an initial Array.
If an argument (number of elements to get) is equal to or higher than the length of an initial Array (number of all elements), then the new Array will be identical to the initial one.
Examples:
[1, 2, "foo", "bar"].last(2)
// Result:
["foo", "bar"]
length()
Returns the number of elements in an Array. This number is called an Array's 'length'.
- Syntax:
Array.length()
- Result:
number
Examples:
[1, 2, "foo", "bar"].length()
// Result:
4
map()
Creates a new Array by performing a provided function on each element of an initial Array.
The new Array will always have the same length as the initial one.
This method uses arrow functions.
- Syntax:
Array.map(function)
- Result:
Array
Examples:
'Only supported by' errors
Be careful:
map()
requires that the provided function can by performed on all of the Array's elements.For example, you can only perform math equations like multiplying if all of the Array's elements are numbers. See example 4.
// Create a new Array with elements multiplied by 2:
[3, 5, 23].map(el => el * 2)
//Result:
[
6,
10,
46
]
[{Answer:1,QuestionId:2}].map(el => el.Answer)
skip()
Creates a new Array that skips the first n elements and consists of the rest of the elements of the initial Array.
If an argument (number of elements to get) is equal to or higher than the length of an initial Array (number of all elements), then the new Array will empty.
Examples:
[1, 2, "foo", "bar"].skip(2)
// Result:
["foo","bar"]
sort()
Creates a new Array with elements sorted in an ascending direction.
- Syntax:
Array.sort()
- Result:
Array
- Similar methods: sortDesc(), sortBy(), sortByDesc()
You can sort Arrays that:
- contain only numbers and Strings representing numbers or
- contain only dates or
- contain only Strings (they will be sorted alphabetically).
Examples:
[1,3,1,2].sort()
// Result:
[1,1,2,3]
sortDesc()
Creates a new Array with elements sorted in a descending direction.
- Syntax:
Array.sortDesc()
- Result:
Array
- Similar methods: sort(), sortBy(), sortByDesc()
You can sort Arrays that:
- contain only numbers and Strings representing numbers or
- contain only dates or
- contain only Strings (they will be sorted alphabetically).
Examples:
[1,1,2,3].sortDesc()
// Result
[3,2,1,1]
sortBy()
Sorts an Array of Objects by one of their properties in an ascending order.
- Syntax:
ArrayOfObjects.sortBy(function)
- Result:
Array
- Similar methods: sort(), sortDesc(), sortByDesc()
You can sort by property values that:
- contain only numbers and Strings representing numbers or
- contain only dates or
- contain only Strings (they will be sorted alphabetically).
Examples:
[{Answer:3,QuestionId:"asd"},{Answer:1,QuestionId:"casd"}].sortBy(el => el.Answer)
// Result:
{
"Answer" : 1,
"QuestionId" : "casd"
},{
"Answer" : 3,
"QuestionId" : "asd"
}
sortByDesc()
Sorts an Array of Objects by one of their properties in a descending order.
- Syntax:
ArrayOfObjects.sortByDesc(function)
- Result:
Array
- Similar methods: sort(), sortDesc(), sortBy()
You can sort by property values that:
- contain only numbers and Strings representing numbers or
- contain only dates or
- contain only Strings (they will be sorted alphabetically).
Examples:
[{Answer: 1,QuestionId:"asd"},{Answer:5,QuestionId:"casd"}].sortByDesc(el => el.Answer)
// Result:
{
"Answer" : 5,
"QuestionId" : "casd"
},{
"Answer" : 1,
"QuestionId" : "asd"
}
sum()
Returns a sum of all of the Array's elements. It only works if all of the elements are numbers.
- Syntax:
Array.sum()
- Result:
number
Examples:
[1, 2].sum()
// Result:
3
unique()
Creates a new Array with unique elements of the initial Array. In other words, this method clears an Array of duplicates.
To be treated as duplicates, two or more elements have to be equal and have the same type. For example, 1
(number) and "1"
(String) will not be treated as duplicates.
[1,1,2,3,"foo","bar","foo", "2"].unique()
// Result:
[
1,
2,
3,
"foo",
"bar",
"2"
]
Arrays of Objects
An Array with elements that are Objects is called an Array of Objects. Most often, these Objects have the same properties. Arrays of Objects are an extremely common way to store and pass data when using JSON format.
In fact, some results of AEL methods are Arrays of Objects, for example nlu.variablesList
, nlu.intentsList
or system.conversation
.
//This is the examplary result of nlu.variablesList
[
{
"name": "time",
"value": {
"grain": "day",
"type": "value",
"value": "2022-06-09T00:00:00.000+02:00",
"values": [
{
"grain": "day",
"type": "value",
"value": "2022-06-09T00:00:00.000+02:00"
}
]
},
"entity": "sys.Time",
"text": "tomorrow"
},
{
"name": "person",
"value": "James",
"entity": "sys.Person",
"text": "James"
},
{
"name": "person",
"value": "Michael",
"entity": "sys.Person",
"text": "Michael"
}
]
Arrow functions
Arrow functions is a concept taken from JavaScript ES6 (learn more).
In Expression language, arrow functions are used in Array methods to:
- define a condition that every item of an Array will be tested against (eg. in
filter()
method), - perform a function on every item of an Array (eg. in
map()
method).
The name "arrow functions" is taken from an arrow =>
used to separate an argument from a function.
Arrow function perform an operation on every element of an Array (iterate through an Array) and return the results.
Every arrow function consists of:
- An argument's name defined by the user. From now on, this name will indicate an element of an Array that is currently processed. This is why they are usually defined as
el
,elem
orelement
. The name of the argument can be anything, though. It doesn't change the way in which a function works. - An arrow
=>
. - A function to be performed on every element of an Array that uses the named argument (eg.
el * 2
).
Examples of an arrow function:
el => el * 2
or elem => elem * 2
This two arrow functions do the exact same thing.
This arrow function will take every element of an Array and multiply it by 2. Such arrow function would be used as an argument of the map()
method, eg. [1, 3, 4].map(el => el * 2)
. The result would be a new Array: [2, 6, 8]
.
To use arrow function to define a condition, it has to return either true
or false
as its result.
For example, the arrow function below is used as an argument for filter()
method:
[2, 14, 22, 17, 5, 8].filter( el => el > 10)
The result of the function el > 10
can be either true
or false
, so it is a correct way to create a condition. Every element of the Array [2, 14, 22, 17, 5, 8]
will be tested against it and the filter()
method will create a new Array containing only the elements that fulfilled the condition el > 10
(its result was true
).
The result of the expression above is [14, 22, 17]
.
Updated 6 months ago
Learn more about Expression language and its methods & APIs: