NLU API
NLU API enables a bot designer to access data recognized by NLU and use it in bot's flows.
Each time a bot designer checks Request user input
in any block, the recognized intents and entities become accessible through nlu
environment, beginning from the next block. For example, to get the name of the recognized intent, a bot designer can input nlu.intent
inside any of the block input fields.
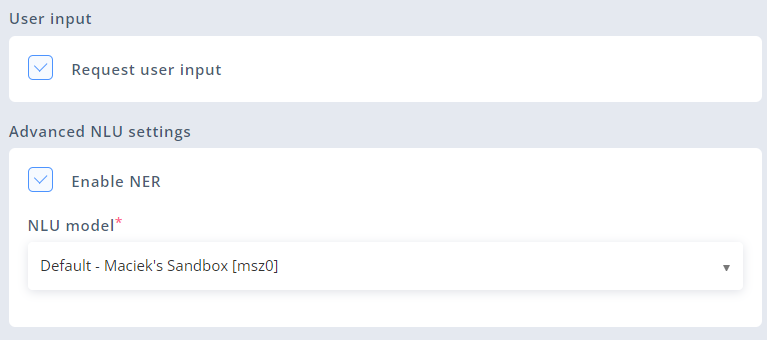
List of NLU properties
Intent property | Description | Type |
---|---|---|
nlu.intent | A name of the main intent that has been recognized. Learn more | String |
nlu.intentScore | A score of the main intent that has been recognized (from 0.01 to 1). Learn more | Number |
nlu.intents | An Object containing all recognized intents with their scores. Learn more | Object |
nlu.intentsList | An Array of Objects containing all recognized intents with their scores. Learn more | Array of Objects |
nlu.hasIntent(intentName) | Returns true if an intent of a given name has been recongized. Learn more | boolean |
Variables and entities
Variables are values or Objects derived from recognized entities. Whenever an entity is recognized by NLU, it can be accessed in flows using properties listed below. Learn more about entities and variables here. API-wise, variables may have different properties, depending on their type. They can be accessed with nlu.variablesList property.
Variable property | Description | Type |
---|---|---|
nlu.variables | An Object containing recognized variables. Learn more | String |
nlu.variablesList | An Array of Objects containing all recognized variables with comprehensive data about each one of them. Learn more | Array of Objects |
Misc property | Description | Type |
---|---|---|
nlu.user_input | Contains a String with the exact text sent by the user. Learn more | String |
nlu.intent
Contains a name of the main intent that has been recognized. The name is defined by a bot designer during NLU development. Learn more about intents here.
// For user input "I want to make a bank transfer."
// Access the recognized intent:
nlu.intent
// The result:
"bank_transfer"
// (This is an examplary intent name that has to be defined first by a bot designer.)
nlu.intentScore
Contains a score of the main intent that has been recognized. The value is a Number from 0.01 to 1. Learn more about intent score here.
// For user input "I want to make a bank transfer."
// Access the intent's score:
nlu.intentScore
// The result:
0.9
// which means that the intent, which we may have defined as "bank_transfer", has a score of 90%
nlu.intents
Contains an Object in which intent names are stored as Object properties and intent scores are stored as Object values.
Multiple intents are detected in one of the following cases:
- A dedicated NLU block appears in FLOW and additional intent classification is performed
- NLU is created as multi-intents and there are training phrases with multiple intents set
Make sure you organization has proper settings set
Organization needs permissions Access to Complex NLU model type & Access to multi-intent NLU. NLU needs to be created as multi-intent.
// For the user input "I need your help in making a bank transfer",
// NLU recognized 2 intents: 'bank_transfer' (score: 0.9) and 'help' (score: 0.2).
// Print nlu.intents:
nlu.intents
// The result:
{
bank_transfer: 0.9,
help: 0.2
}
// For the user input "I need your help in making a bank transfer",
// NLU recognized 2 intents: 'bank_transfer' (score: 0.9) and 'help' (score: 0.2).
// Access the score of the 'bank_transfer' intent:
nlu.intents.bank_transfer
// The result:
0.9
nlu.intentsList
Contains an Array of Objects. Each Object represents one recognized intent and has two properties: name and score.
Multiple intents are detected in one of the following cases:
- A dedicated NLU block appears in FLOW and additional intent classification is performed
- NLU is created as multi-intents and there are training phrases with multiple intents set
Make sure you organization has proper settings set
Organization needs permissions Access to Complex NLU model type & Access to multi-intent NLU. NLU needs to be created as multi-intent.
// For the user input "I need your help in making a bank transfer."
// NLU recognized 2 intents: 'bank_transfer' (score: 0.9) and 'help' (score: 0.2).
// Print nlu.intentsList:
nlu.intentsList
// The result:
[
{
name: "bank_transfer",
score: 0.9
},
{
name: "help",
score: 0.2
}
]
// For the user input "I need your help in making a bank transfer."
// NLU recognized 2 intents: 'bank_transfer' (score: 0.9) and 'help' (score: 0.2).
// Access the score of the first recognized intent:
nlu.intentsList[0].score
// The result:
0.9
nlu.hasIntent(intentName)
Returns true if an intent of given name has been recongized.
// For the user input: "I need your help in making a bank transfer."
// NLU recognized 2 intents: 'bank_transfer' (score: 0.9) and 'help' (score: 0.2).
// Check if 'help' intent has been recognized:
nlu.hasIntent("help")
// The result:
true
// For the user input: "I need your help in making a bank transfer."
// NLU recognized 2 intents: 'bank_transfer' (score: 0.9) and 'help' (score: 0.2).
// Check if 'stolen_card' intent has been recognized:
nlu.hasIntent("stolen_card")
// The result:
false
// For the user input: "I need your help in making a bank transfer."
// NLU recognized 2 intents: 'bank_transfer' (score: 0.9) and 'help' (score: 0.2).
// Check if at least one of these intents have been recognized: 'stolen_card', 'bank_transfer'
nlu.hasIntent("stolen_card") OR nlu.hasIntent("bank_transfer")
// The result:
true
nlu.variables
Contains an Object in which variable's names are stored as Object properties and variable's values are stored as Object values.
//For user input: "My name is Kate, my email is [email protected]"
//Print the whole Object:
nlu.variables
//Result:
{
"person": "Kate",
"email": {
"type": "value",
"value": "[email protected]"
}
}
//For user input: "My name is Kate, my email is [email protected]"
//Access the 'person' variable:
nlu.variables.person
//Result:
"Kate"
//For user input: "My name is Kate, my email is [email protected]"
//Access the 'email' variable's value:
nlu.variables.email.value
//Result:
"[email protected]"
//For user input: "John is from the United States and Maria is from Brazil."
//Print the whole Object:
nlu.variables
//Result:
{
"country" : "United States",
"forename" : "John"
}
If you try to call the variable that hasn't been found, it will return null
, eg. for the user input "I lost my credit card" nlu.variables.person
will return null
.
Limitations
nlu.variables
property is an Object that contains only 2 variable's properties:name
andvalue
. To access all variable's properties, usenlu.variablesList
- When more than one variable derived from the same entity is recognized, only first one (occurring earlier in the text) will be accessible through
nlu.variables
. To access absolutely all recognized variables, usenlu.variablesList
. E.g. for the user input "Kate and Monica",nlu.variables.person
will returnKate
.
nlu.variablesList
Contains an Array of Objects. Each Object represents one of the recognized variables and consists of all of its properties. Every variable has at least 4 properties: name, value, entity and text. Variables derived from regex entities have 2 more: match and groups. Value property may be either a String or an Object, especially in case of variables derived from system entities (see System entities properties below)
Following table contains all of the properties that may be found in nlu.variablesList Objects:
Property | Entity type | Type | Description |
---|---|---|---|
name | All | String | A name of the variable, usually equal to the value of entity . |
value | All | String or Object | A processed value of the variable, eg. formatted date, or an Object with more specific information (see Variables from system entities) |
entity | All | String | An entity's name that the variable is referring to. |
text | All | String | A raw, unprocessed part of the user input that has been recognized as an entity. Eg. 'tomorrow' that will be recognized and formatted as a date. Known issue: in some cases, text variable may gather additional characters if there is no whitespace between entity and them, eg. for user input "2f", numeral variable will have value = 2 , but text = 2f . |
match | Regex | String | A part of the String matched by a regular expression. |
groups | Regex | Object | One or more defined groups captured by a regular expression. |
//For user input: "Twenty three"
//Print the whole Array:
nlu.variablesList
//Result:
[
{
"name": "numeral",
"value": {
"type": "value",
"value": 23
},
"entity": "sys.Numeral",
"text": "twenty three"
}
]
//For user input: "James will meet Michael tomorrow."
//Print the whole Array:
nlu.variablesList
//As we can see below, the result is quite long.
//This is because NLU recognized 2 person entities (James, Michael)
//and 1 time entity (tomorrow), which is interpreted as time.
//Also, time variable has additional property: 'values'.
//(learn more about time variables below)
//Result:
[
{
"name": "time",
"value": {
"grain": "day",
"type": "value",
"value": "2022-06-09T00:00:00.000+02:00",
"values": [
{
"grain": "day",
"type": "value",
"value": "2022-06-09T00:00:00.000+02:00"
}
]
},
"entity": "sys.Time",
"text": "tomorrow"
},
{
"name": "person",
"value": "James",
"entity": "sys.Person",
"text": "James"
},
{
"name": "person",
"value": "Michael",
"entity": "sys.Person",
"text": "Michael"
}
]
//For user input: "Twenty three and 45"
//Create an Array of values of all the recognized numeral (system) variables using Array's methods .filter() and .map()
nlu.variablesList.filter(el => el.name = "numeral").map(el => el.value.value)
//Result:
[
23,
45
]
//Tip: go to Arrays page to learn more about .filter() and .map()
nlu.user_input (deprecated - use system.userInput
)
system.userInput
)Contains a String with the exact text sent by the user.
// For the user input "Hello World"
//Get the user input:
nlu.user_input
// The result:
"Hello world"
see also system.userInput.
Using NLU API in blocks fields - examples
This section provides a few examples of using NLU API in blocks.
You can use NLU API in any of the block's input fields that expect Expression language or JavaScript (eg. Responses, Memory, Output Conditions, Commands).
Steering conversation with Output Conditions
In the example below, if the intent "bank_transfer" was recognized, the bot will steer the conversation using "Go to bank transfer" Output.
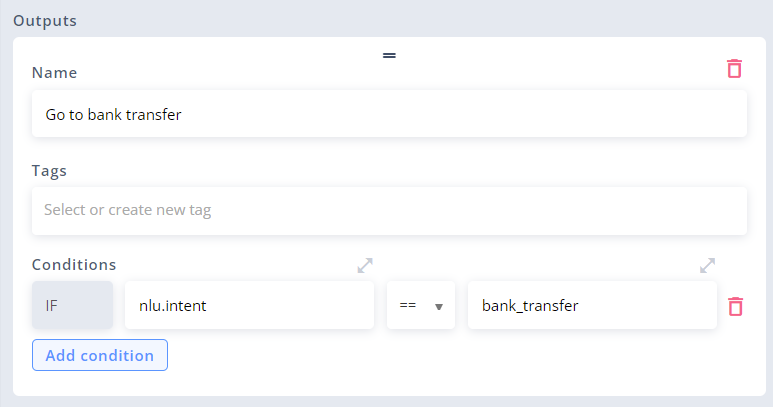
Example - Steering conversation with Outputs' Conditions
Printing NLU results in Responses
You can use the information gathered with NLU by calling it directly in Responses input field in a form of interpolation.
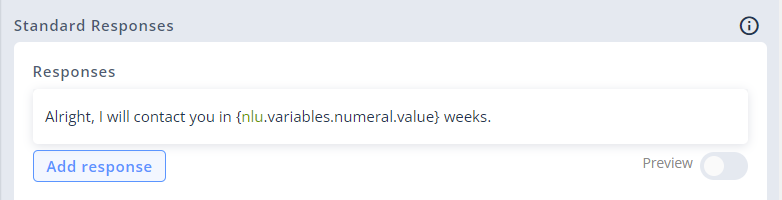
Example - Printing NLU results in Responses
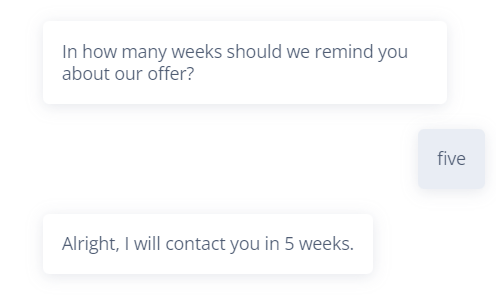
Result - Printing NLU results in Responses
Saving NLU results to Memory
You can use memory to save your current NLU results for later use. The example below shows how to save all available NLU results to memory.intents_1 and memory.variables_1.
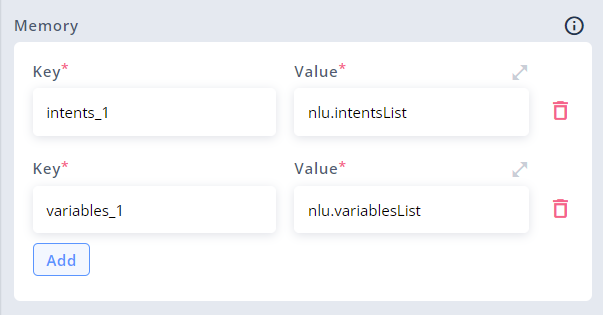
Saving all NLU results to Memory
You can also use Memory to create one Object to store all of the current NLU results there. Let's call this Object nlu_results_1
:
// Memory input field
// Key:
nlu_results_1
//Value:
{
"intents": nlu.intentsList,
"variables": nlu.variablesList
}
// This is how you can later obtain the list of variables stored in it:
memory.nlu_results_1.variables
NLU data lifespan
Remember, each time a bot requests user input during the conversation, previous NLU data gets overwritten!
To access NLU results from an earlier user input, you have to first save it to memory before it gets overwritten, just like in the examples above.
Comparing NLU results with external data in Memory
Many times, you will want to compare specific information requested from the user with data previously stored in Memory, eg. obtained from an API request to external database.
In the example below, we will check if the date recognized by NLU (which is the value of 'time' variable) is equal to the birthdate obtained from API response (see: Integrations).
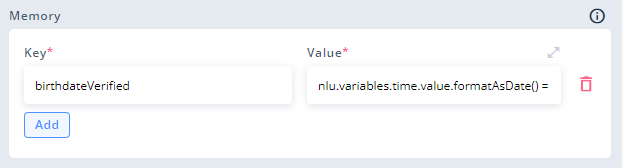
Comparing NLU results with external data in Memory (full snippet below)
// Memory input field
// Key:
birthdateVerified
// Value:
nlu.variables.time.value.formatAsDate() = memory.response.birthdate
// The result of
memory.birthdateVerified
// when the dates are equal and in the same format:
true
Processing NLU results with JavaScript
As it is with every Automate environment, you can use NLU API properties directly in JavaScript code. Learn about using JavaScript here.
In the following example, we use JavaScript's keyword typeof
to check the type of a value stored in nlu.variablesList:
typeof nlu.variablesList
// Result:
'Object'
// More specifically, this is an Array, but in JavaScript, every Array is technically an Object.
typeof nlu.intent
//Result:
'String'
Selected variables derived from system entities
This section provides information and use cases for variables derived from selected system entities that have special properties.
System entities are the entities built-in into every Automate bot. Learn more about system entitites here.
Language support and system entities
Available system entities may vary based on the language of the bot. See Language support for more information.
time
Recognizes date and time.
Characteristic value properties:
value
- date value as a String, eg.2022-06-09T00:00:00.000+02:00
grain
- determines the level of the date's accuracy, gets one of these values:hour
,day
,month
oryear
(see example 3)values
- an Array of three Objects of the same prototype asvalue
. They store multiple interpretations of the same user input recognized by NLU astime
. For example, the phrase "on Friday" will have the dates of the next 3 Fridays stored in there.
Learn more about time entity here.
Learn more about using and manipulating dates on DateTime API page.
// For user input: "tomorrow" (it was 8th of June when we wrote this)
[
{
"name" : "time",
"value" : {
"grain" : "day",
"type" : "value",
"value" : "2022-06-09T00:00:00.000+02:00",
"values" : [
{
"grain" : "day",
"type" : "value",
"value" : "2022-06-09T00:00:00.000+02:00"
}
]
},
"entity" : "sys.Time",
"text" : "tomorrow"
}
]
duration
Recognizes the duration of time.
Characteristic properties:
unit
- determines the unit of time, eg. 'year', 'week' or 'day'unit_name
- another way of presenting unit and value, where the property name is a unit, and a value is a Number stating the durationnormalized
- an Object containing two properties:unit
(which value is always 'value') andvalue
which is the duration given in seconds
// Get information on duration for the user input "2 weeks":
nlu.variables.duration
// The result:
{
"year": 2,
"normalized": {
"unit": "second",
"value": 63072000
},
"unit": "year",
"type": "value",
"value": 2
}
url
Recognizes urls.
Characteristic value property:
domain
- determines a domain of the recognized url
// Get a domain for the user input "https://sentione.com/features/automate/"
nlu.variablesList.value.domain
// The result:
"sentione.com"
Updated 29 days ago
Learn more about Expression language and its methods & APIs: