Carousel
Feature Description
Carousel is an interactive element of the webchat interface that allows presentation of content in the form of a horizontal slider with multiple slides. Each slide can contain a title, text, graphics, and action buttons. Users can navigate between slides using buttons or touch gestures (swipe).
Applications
Carousel is an ideal solution for:
- Presenting products or services
- Displaying multiple options to choose from
- Creating interactive guides
- Presenting multistep instructions
- Showcasing image galleries with descriptions
Carousel Implementation
There are two ways to implement a carousel in SentiOne webchat:
- Using the Carousel block - a simpler, visual method available in the Automate interface
- Using the Carousel command - an advanced method requiring knowledge of JSON format
1. Implementation using the Carousel Block
SentiOne Automate offers a dedicated Carousel block that allows easy creation of carousels without the need to manually write JSON code.
Step 1: Adding the Carousel Block to Flow
- In the Flow module, open modal with all block types
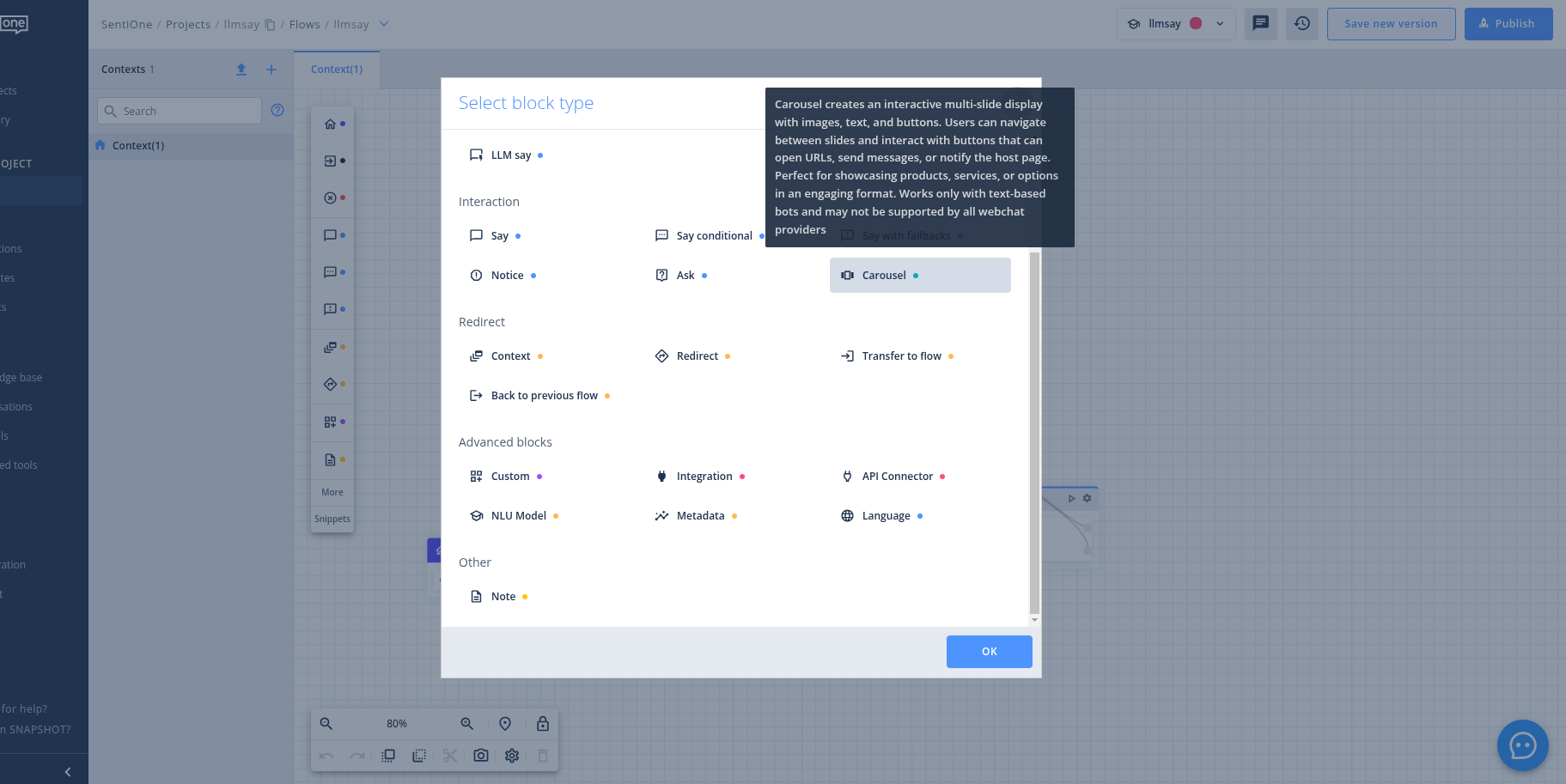
- In the "Interaction" category, select the "Carousel" block
Step 2: Configuring the Carousel Block
After adding the Carousel block to Flow, you can configure its parameters:
- Name the carousel
- Specify whether the carousel should respond to user input
- In the "Preview of carousel" section, you'll see a preview of the carousel being created
- In the "Slide" section, you can configure individual slides:
- Slide title
- Image URL and alternative text
- Slide content (with Markdown formatting support)
- Buttons with different action types
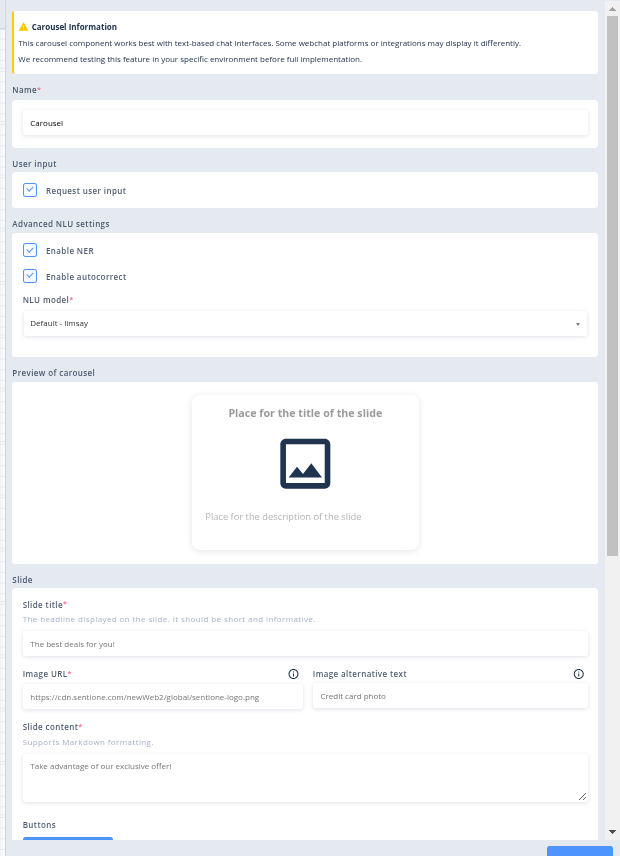
Button Action Types
The carousel supports three types of buttons, each with its own actions:
-
Open URL - opens the specified URL in a new browser tab
- Requires providing a URL that will be opened when the button is clicked
-
Send Reply - automatically sends a specified message to the webchat, as if it was typed by the user
- Requires providing the content of the message that will be sent
-
Notify Host - sends a notification to the application hosting the webchat, enabling interaction between the carousel and the page on which the webchat is embedded (read more below) Read more
- Requires providing a button identifier (buttonId) that will be passed to the event handler function
Customizing Carousel Appearance with customCss
SentiOne webchat allows full customization of the carousel appearance using the customCss
mechanism. This enables you to adapt the visual presentation of the carousel to your visual identity, including image size and scaling.
Configuration in Channels Module
Before you can use custom styles, you need to configure them in the Channels module. As shown in the screenshot, there is a dedicated section for custom styling under "Custom Styles" where you can:
- Set a Chat Custom Styles URL
- Add direct CSS code in the Chat Custom Styles CSS field
This configuration panel allows you to apply custom styling to various elements, including the carousel component.
Legacy mode implementation (without using Channels module - only for Advanced users)
If you're using Legacy Mode for embedding the webchat, you must use the customCSS mechanism through the appearance.styles
property structure:
window.sentiOneChat.initWebChat({
// Other configuration parameters...
appearance: {
styles: {
// URL to an external stylesheet
url: 'https://cdn.sentione.com/chat-ui-launcher/themes/dark-blue.css',
// Custom CSS rules for carousel elements
customCss: `
.carousel {
border-radius: 10px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
}
.carousel__container img {
object-fit: cover;
width: 100%;
height: 200px;
}
.carousel__message {
padding: 12px;
background-color: #f5f5f5;
}
.carousel .button.primary {
background-color: #4caf50;
border-color: #4caf50;
}
`,
// Main theme color
themeColor: '#4caf50'
}
}
});
Most Commonly Customized Carousel Elements
CSS Element | Description | Example |
---|---|---|
.carousel | Main carousel container | border-radius: 10px; |
.carousel__container | Container with slides | max-width: 450px; |
.carousel__container img | Images in the carousel | object-fit: contain; height: 220px; |
.carousel__arrows | Navigation buttons | background-color: rgba(0,0,0,0.5); |
.carousel .button.primary | Main action button | background-color: blue; |
.carousel .button.secondary | Additional buttons | border-color: gray; |
This mechanism gives you complete control over the carousel presentation, allowing you to adapt it to your design needs and ensure a consistent user experience that aligns with your brand's visual identity.
2. Implementation using the Carousel Command (advanced)
For advanced users, SentiOne enables adding a carousel through direct implementation of a JSON command in the "Say" node in the Flow module:
- In the "Commands" section, add a command named
Carousel
- In the "Params" field, enter a JSON array with slides:
[
{
"title": "Slide title",
"content": {
"text": "Slide description",
"graphics": {
"url": "https://example.com/image.jpg",
"alt": "Image description"
},
"buttons": [
{
"actionType": "open_url",
"text": "Visit website",
"actionParams": {
"url": "https://example.com"
}
}
]
}
}
]
Handling notify_host Events in the Host Application
The notify_host
type buttons allow for communication between the carousel in the webchat and the page on which it is embedded. To handle the click events of these buttons, you need to implement an appropriate handler in the page code.
Example Implementation of Carousel Event Handling
The following HTML code demonstrates an example implementation of a page with an embedded webchat that handles events from notify_host
type buttons in the carousel:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Example of Carousel Integration with Your Own Page</title>
</head>
<body>
<!-- SentiOne Webchat Script -->
<script src='https://cdn.sentione.com/chat-ui-launcher/chat.1.60.2.js' type='application/javascript'></script>
<script type="text/javascript">
// Webchat initialization
window.sentiOneChat.initWebChat({
chatSrc: "https://webchat.your-environment.chatbots.sentione.com/fullscreen",
channelId: "your-channel-id",
// Handler for carousel button click events
onCarouselButtonClicked: function (button) {
console.log('Carousel button clicked:', button);
// Checking the button ID and performing appropriate actions
if (button.id === "create_ticket") {
// Example action - opening a problem report form
openTicketForm();
} else if (button.id === "show_products") {
// Example action - navigating to the product list
navigateToProducts();
}
// You can also use button.actionParams.buttonId if you're passing additional parameters
}
});
// Example functions called after button clicks
function openTicketForm() {
// Code responsible for opening the report form
alert('Opening problem report form');
// document.getElementById('ticketForm').style.display = 'block';
}
function navigateToProducts() {
// Code responsible for navigating to the product page
alert('Redirecting to product list');
// window.location.href = '/products';
}
</script>
</body>
</html>
Parameters of the onCarouselButtonClicked
Event
onCarouselButtonClicked
EventThe onCarouselButtonClicked
function receives as an argument a button object (button
) that contains all information defined in the Carousel command:
{
actionType: "notify_host", // Action type (always "notify_host" for this event)
text: "Button text", // Text displayed on the button
actionParams: {
buttonId: "uniqueButtonId" // Additional parameter passed to the handler
}
}
Practical Applications
notify_host
events can be used for:
- Integration with external systems - e.g., opening forms, transferring data to CRM
- Personalization of user experience - customizing page content based on choices in the carousel
- Tracking user behavior - analytics of clicks and interactions with the carousel
- Initiating business processes - e.g., starting the order placement process
Tips and Best Practices
-
Optimal number of slides - For the best user experience, it is recommended to use 2 to 5 slides in a carousel.
-
Images - Use clear, attractive graphics that represent your content well.
-
Text length - Remember that too long titles and button texts will be automatically shortened and ended with an ellipsis (...). To ensure optimal appearance:
- Slide titles should have a maximum of 40-50 characters
- Button texts should not exceed 20-25 characters
- Slide content should be concise and readable
-
Testing - Remember to check the operation of the carousel on different devices to ensure an optimal experience for both mobile and desktop users.
-
Consistency - Ensure that all slides in the carousel have a similar structure and style, which will make navigation easier for users.
Updated about 2 months ago