Strings
A String is one of the data types in AEL (Expression language).
A String is a sequence of one or more characters that may consist of letters, numbers, or symbols. A string can contain any sequence of characters, visible or invisible, and characters may be repeated.
In other words, a String is a plain text.
In AEL, Strings are contained within double quotation marks.
Examples of Strings:
- "Robert California"
- "33 times"
- "$30 for you."
- "["
- "@#$"
- "{this is not an object nor expression, it's just a string, because it's contained in quotation marks}"
In Automate, Strings can be found in any of the input fields, eg. Responses, output conditions, or values saved to Memory.
List of String methods
Method | Description |
---|---|
Concatenate | Joins multiple Strings by using the + operator. |
replace(old,new) | Allows you to replace some parts of a String. |
toDateTime(format) | Converts a String into DateTime value. |
toLower() | Changes all characters to lower case. |
toUpper() | Changes all characters to upper case. |
toNumber() | Converts a String into a Number (either round number or with decimal points). |
length() | Returns length (number of characters) of a String. |
contains(searchString) | Determines whether or not the String includes searchString . |
slice(indexStart, indexEnd) | Returns a new String containing the extracted section of the String. |
Strings in Responses
The whole input of the Response is a String by default, so you don't need to contain it in quotation marks.

Plain text in Responses is a String, but it doesn't require quotation marks.
Interpolation (adding variables and AEL to Responses)
To interpolate (add) a variable or an expression into a Response, use curly brackets {}
.

Current time is interpolated into the Response.
Escaping {}
{}
To print out { } in the Response instead of using Interpolation, you have to use escape character \
before each character you want to escape.

This Response will print out this message: "Objects are contained in curly brackets: {}."
Strings in Input fields
Strings in input fields and in AEL expressions have to be contained within a pair of double quotation marks ""
. Single quotation marks will cause an error (Expression is not valid
).
Quotation marks can be omitted when an input field consists of nothing but a String that doesn't contain any whitespaces or special characters.
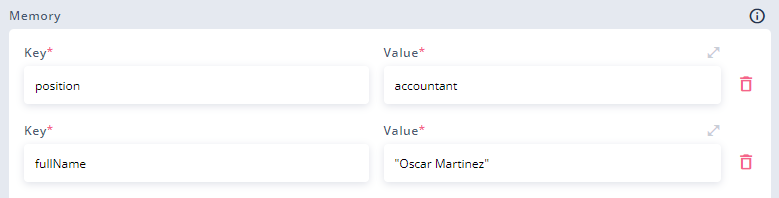
memory.position
doesn't have any whitespaces nor special characters, so it's a valid string.
memory.fullName
contains a whitespace, so it needs quotation marks.
String methods descriptions
Concatenate
Join multiple Strings together by using conatenation operator +
.
// For:
// memory.name = "Adam"
// memory.amount = 100
"I would like to make a transfer to " + memory.name + " for the amount of $" + memory.amount + "."
//Result:
"I would like to make a transfer to Adam for the amount of $100."
replace(old,new)
Replace a part of the String with the new String.
"Hello people".replace("people", "world")
//Result:
"Hello world"
toDateTime()
Converts a String into a DateTime value. More info here.
"2010-06-30T01:20+02:00".toDateTime()
//Result:
"2010-06-30 01:20"
toLower()
Change all of the characters to lower case.
"HeLLo WoRLd".toLower()
//Result:
"hello world"
toUpper()
Change all characters to upper case.
"hello world".toUpper()
//Result:
"HELLO WORLD"
toNumber()
Converts a String into a Number.
"10.5".toNumber()
//Result:
10.50
length()
Returns length (number of characters) of the String.
"Automate".length()
//Result:
8
contains(searchString)
Performs a case-sensitive search to determine if searchString
can be found inside the String. Returns true
if found, otherwise it returns false
.
"Automate is awesome!".contains("Automate") //Result: true
"Automate is awesome!".contains("SentiOne") //Result: false
slice(indexStart, indexEnd)
Returns a new String containing the extracted section (up to indexEnd
, which is optional) of the string. If indexEnd
is not set, the returned String contains characters from indexEnd
till the end of the String.
This method works in the same way as JavaScript's slice method, so please refer to it's documentation.
"Automate is awesome!".slice(0, 8) // Result: "Automate"
"Automate is awesome!".slice(12) // Result: "awesome!"
"Automate is awesome!".slice(-8) // Result: "awesome!"
Updated over 1 year ago
Learn more about Expression language and its methods & APIs: