AEL overview
Automate Expression language (AEL) is a simple, user-friendly, custom language developed by SentiOne.
AEL lets users write simple, one-line expressions (units of code) that return a result value. AEL is mainly used in flow's blocks.
The result value can be one of the following data types:
👉 Learn more about data types in AEL
AEL was originally inspired by JavaScript and shares many features with it.
Despite the fact that Expression language was originally inspired by JavaScript, non-programming users can easily learn how to use it.
What you can do with AEL
The following list is a general scope of what can be done with AEL in Automate:
- Accessing properties stored in Memory, responses from external APIs, Knowledge Base, Extra data and built-in APIs (NLU API, System API, System Math API, DateTime API).
- Creating, processing and filtering data (strings, arrays, objects).
- Creating
if...else
statements; setting up conditions using operators. - Doing math operations; getting current time; processing date and time.
Examples
// Bot's response input:
User input: {system.userInput}
Recognized intent: {nlu.intent}
// Such response may print something like that (depending on the user input and NLU training):
User input: I want to block my credit card.
Recognized intent: block_card
// Save an object to Memory by setting literal object as value, eg.:
{
firstName: "Michael",
lastName: "Scott",
position: "regional manager"
}
// Filter an array so that the new array has only elements larger than 3:
[1, 2, 4, 6].filter(el => el > 3)
// Result:
[4, 6]
if (nlu.variables.time != null) "Thank you for providing the time of your arrival." else "It didn't sound like time to me."
👉 You can learn about available methods, APIs and properties on the following pages of the Expression language section.
Methods and APIs of AEL
Expression language provides a set of methods and built-in APIs that are used to access and process various data.
Methods are built-in functions that can be used on particular data types:
AEL APIs are built-in APIs providing an access to Automate environments and system variables:
👉 Go to Methods and APIs overview page to learn how to use them
Where you can use AEL
The most common place to use AEL are blocks. Generally, there are two types of block fields that accept AEL:
- Responses
- Input fields
There are slight differences between them when it comes to adding values and using AEL expressions inside them.
AEL in Responses
You can use AEL in a Response field of any block by adding an expression within curly brackets {}
.
You can add as many expressions as you need inside one Response.
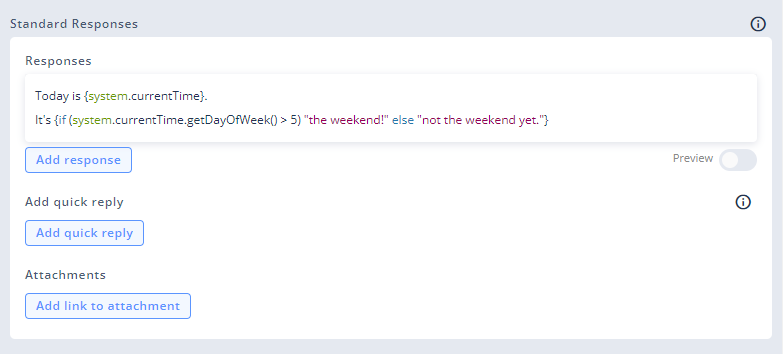
AEL used twice in one Response: to print out the current date and to print out the result of if...else statement
Quick replies
Quick replies are the only block's input fields in which writing and using AEL works exactly the same as in Responses.
AEL in input fields
You can also use AEL and JS in most of the blocks' input fields. You can recognize those fields by the icon above them that opens a multi-line editor:

Multi-line editor icon
Unlike in Responses, you don't need curly brackets {}
to use AEL in input fields. The whole input value is treated as an AEL expression.
The following list contains all of the blocks' input fields that accept AEL expressions:
- Memory values in the Custom blocks
- Conditional statements of the Outputs and Conditional responses (on both sides)
- Commands parameters
- Integration input parameters
- Metadata item values
- Quick replies (* but you need to use
{}
in them)
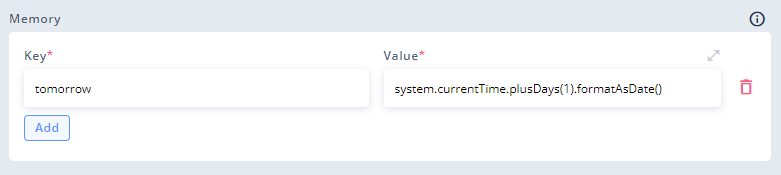
AEL used in Memory to define the value of memory.tomorrow
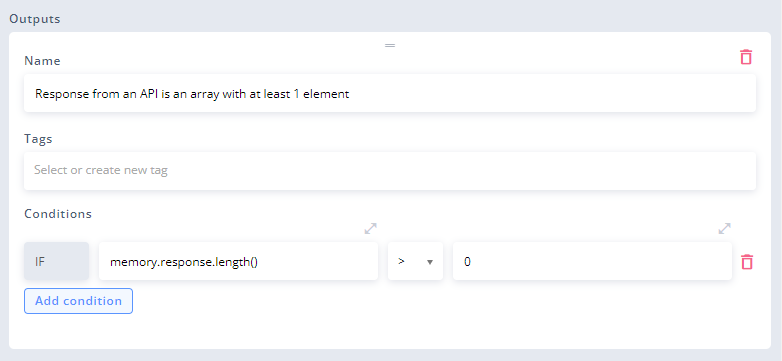
AEL used in output condition (left side)
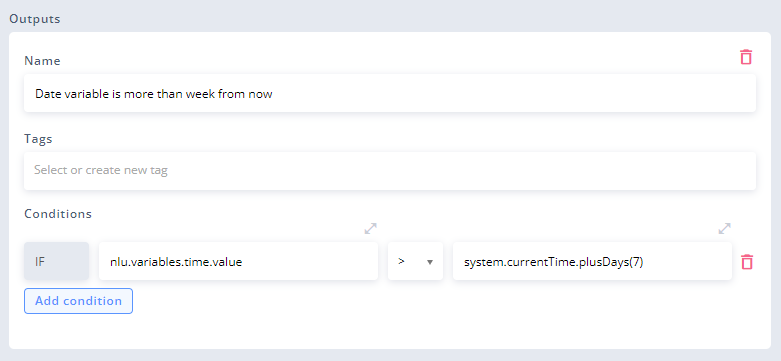
AEL used in output conditions (both sides)
AEL in other places
You can also use AEL in some of the input fields outside of Flows, in the following modules:
General rules of AEL syntax
Overall, AEL's syntax is derived from JavaScript, but there are certain limitations and differences.
The following list contains some of the syntax rules of AEL, including those that are different from JavaScript. Consider them when troubleshooting:
- AEL is a one-line language. New lines will be ignored and an expression will still work - as long as it meets other requirements (like required spaces).
- Operators don't require whitespaces, and more than one whitespace will be ignored. All of these expressions will work:
1 + 3
,1+3
,1+ 3
. - Strings in input fields and in AEL expressions are contained within a pair of double quotation marks. Single quotation marks will cause an error (
Expression is not valid
). - Quotation marks can be omitted when an input field consists of nothing but a string that doesn't contain any whitespaces or special characters.
- In Responses, quotation marks are treated as any other character. You don't have to enclose a Response text with them nor escape them when they are inside the response.
- Expressions in input fields can't end with a whitespace. This will cause en error (
Expression is not valid
).
Common AEL errors
The following list describes the most common AEL errors as well as some tips to handle them:
Expression is not valid
It is caused by a mistake in expression's syntax. It prevents block from being saved. Check your expression against typos and General rules of AEL syntax. Example:"Jim" + "Halpert
.Couldn't get value for index 1. Array size is 0 and array index starts from zero, so maximum index is -1;
Such errors are caused by trying to access an element of an array that doesn't exists. Learn more about arrays here.
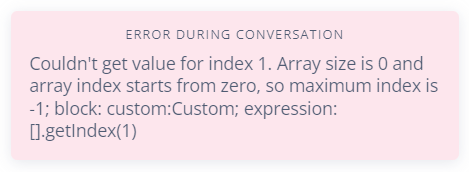
Error messages during conversation contain the exact block and the expression that caused an error
Infinite loop detected
Infinite loop is the situation in which bot's flow keeps returning to the same point, so the operation continues to run endlessly. Debug and avoid them by turning Request User Input on.
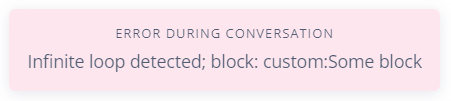
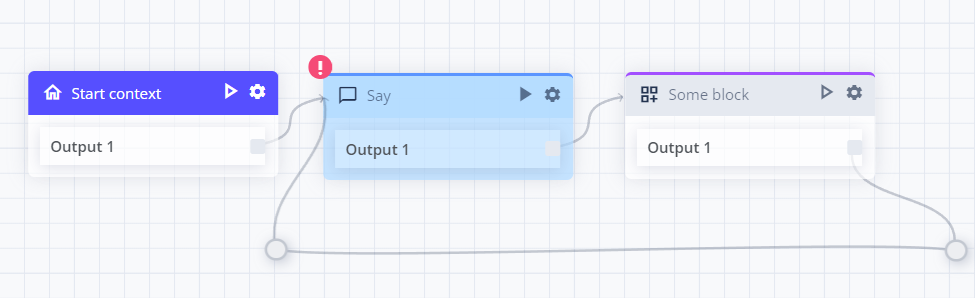
Most basic example of an infinite loop
Operation getIndex() is not supported by type StringValue
This and similar errors are caused by trying to perform an operation that is no allowed by the data type.
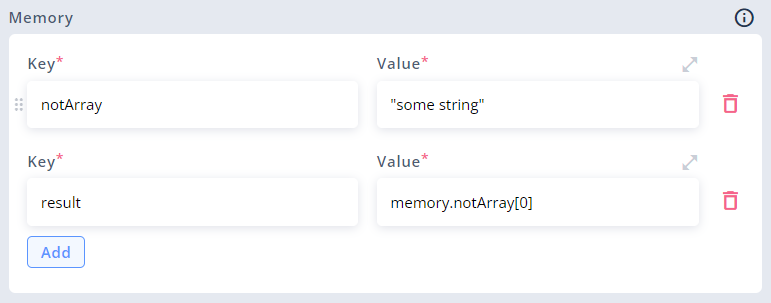
In the example above, memory.notArray
is a string and not an array, so [0] (or getIndex())
operation is not allowed by it, memory.result
will cause an error:
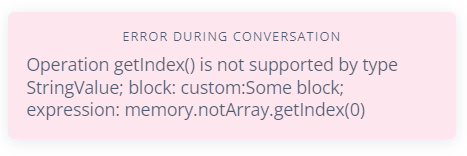
Not comparable values
This error is caused when you try to compare two values that don't have the same type, eg. system.currentTime > "1990-10-10"
. The first one has DateTime type, and the second one - string type.
AEL and JavaScript
AEL has a lot in common with JavaScript. It uses a simplified version of JS syntax and some of its concepts, such as bracket and dot notations, one-line arrow functions, if statements, and a selection of methods to be used on strings, arrays and objects.
What's more, whenever AEL is not enough, you can use JavaScript instead. This is why you should consider learning the basics of JS when developing more advanced bots in Automate.
Updated about 2 years ago
Learn more about writing AEL expressions or go straight to methods and APIs pages to check out examples.