Announcement Banner
Notify user about some important event
Announcement Banner Feature for Web Chat
Overview
The Announcement Banner feature provides a customizable topbar in the web chat interface. It can be configured to display important messages or announcements with options for markdown content, link behaviors, and dismissible actions.
Configuration Options
The announcement banner can be controlled via the configuration object or dynamically triggered using a Command
.
Configuration Object
The announcementBanner
field in the configuration object allows you to predefine a banner for the web chat.
announcementBanner?: {
content: string; // Required. The text or markdown content to display in the banner.
closable?: boolean; // Optional. If true, displays a close button for the banner. Default: true.
markdownLinkBehavior?: 'new-tab' | 'callback'; // Optional. Defines how markdown links behave. Default: 'new-tab'.
onlyOnce?: boolean; // Optional. If true, the banner is shown only once per conversation. Default: true.
};
Example
const config = {
announcementBanner: {
content: "**Welcome!** Click [here](https://example.com) for details.",
markdownLinkBehavior: 'callback',
}
};
Callbacks
You can define callbacks to handle user interactions with the banner:
onAnnouncementBannerLinkClick
: Triggered when a link in the banner is clicked.onAnnouncementBannerLinkClick?: (data: object) => void;
onAnnouncementBannerClose
: Triggered when the banner is closed.onAnnouncementBannerClose?: (data: object) => void;
Dynamic Command
The AnnouncementBanner
command allows dynamic control over the banner during a session. This is particularly useful for context-specific messages.
Command Model
{
content: string; // Required. The text or markdown content to display.
closable?: boolean; // Optional. If true, displays a close button for the banner.
markdownLinkBehavior?: 'new-tab' | 'callback'; // Optional. Defines link behavior.
show?: boolean; // Optional. If false, hides the banner immediately.
}
Example Command
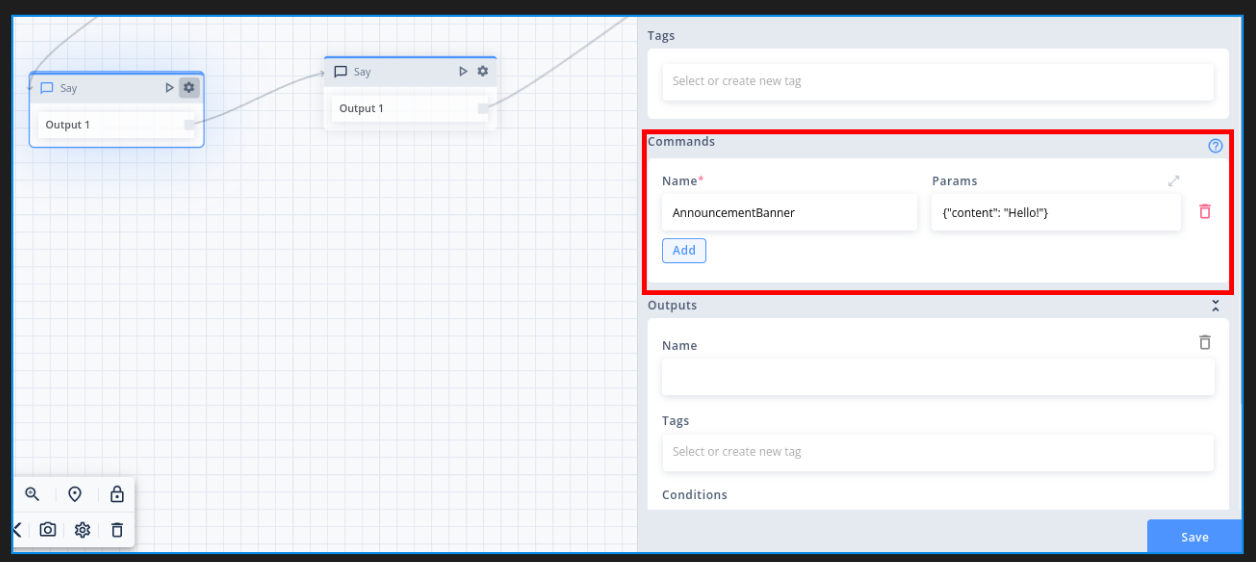
Channel Configuration
You can also define a callback function directly in the channel configuration:
<script type="text/javascript">
window.sentiOneChat.initWebChat({
chatSrc: "ENV_CHAT_SRC",
channelId: "CHANNEL_ID",
onAnnouncementBannerLinkClick: (data) => {
console.log('User clicked link', data)
},
});
</script>
Behavior and Scenarios
General Behavior
- The banner is displayed at the top of the web chat interface when configured or triggered by a command.
- If
closable
is set totrue
, users can dismiss the banner using the close button.
onlyOnce
Setting
onlyOnce
Setting- If
onlyOnce
istrue
, the banner will not reappear after being minimized or after page refresh during the same conversation. - The banner reappears for new conversations or when the web chat is reset.
Special Cases
- If the banner is closed from the configuration object and a new command triggers a banner, the new banner appears regardless of the
onlyOnce
setting. - If the page is refreshed, the banner from the configuration object reappears.
- Commands with
show: false
immediately hide the banner, overriding other settings.
Markdown Link Behavior
new-tab
: Opens links in a new browser tab.callback
: Triggers theonAnnouncementBannerLinkClick
callback, allowing custom handling of link clicks.
Customization
You can style the banner using the customCss
or external CSS file specified in the appearance
field of the configuration object:
appearance: {
styles: {
customCss: ".announcement-banner { background-color: #f0f0f0; color: #333; }"
}
}
Updated about 1 month ago