Arrays
Learn using arrays by creating simple bot projects
Short introduction to arrays
An array is a list of elements.
This is an array: ["Pam", "Angela", 1, true, null, memory.someValue, system.userInput, nlu.intent]
This is also an array: []
This is an array as well: [null]
An array is an ordered list of values. Each value is called an element, and each element has a numeric position in the array, known as its index.
Element of an array can by anything.
An element can be any type of data, eg. a string, a boolean, a number, or even an object or another array. An element can also be a dynamic value, eg. system.userInput
. Each element can have a different type.
Square brackets []
are an array’s trademark.
An array is contained within two square brackets: []
, and its elements are separated by comas. For example, this array: ["one", 1]
contains two elements: the string "one"
and the number 1
.
Need a list of numbers, dates or anything else? Use an array.
Arrays are commonly used to store, process and send data. In Automate, you may meet them while using NLU API, Knowledge Base, integrations with external databases etc.
In Automate, you can create, access, and modify arrays using Expression language (AEL) or JavaScript (JS).
Create Array in application
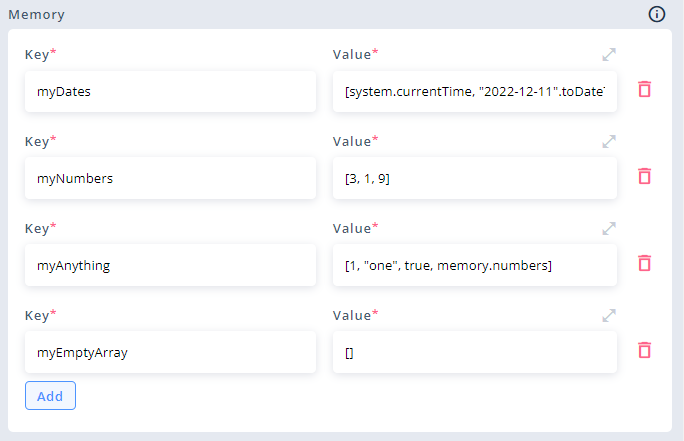
Examples of how an Array can be created and stored in Memory
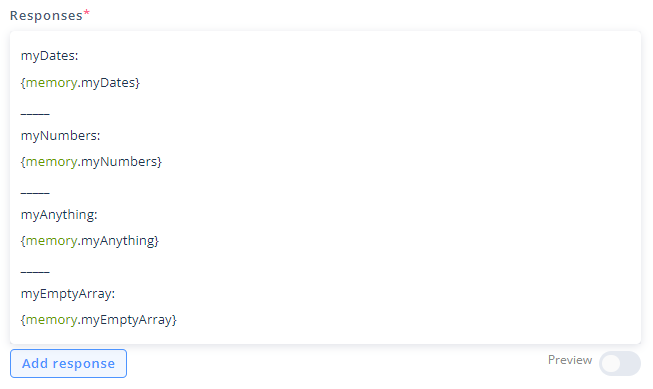
You can print the whole array in a Response.
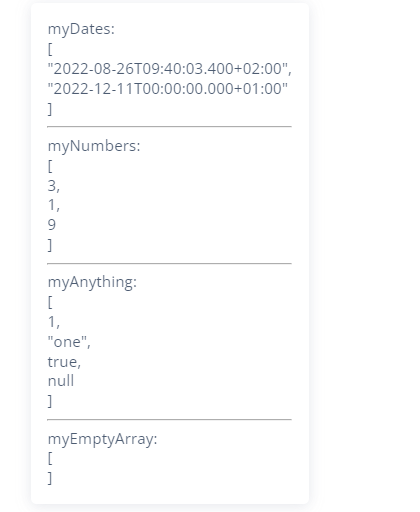
Example of how the output will look
You can access and print an array’s element using its index.
- Index is a number stating position of the element in an array.
- To access an element by its index, add it directly after an array, wrapped in square brackets, eg.
[3]
. - The first element of an array has index 0. So to access the first element of an array, use
[0]
Example 1:
// Access the fourth element of an array:
[1, 2, "foo", "bar"][3]
// Result:
"bar"
Example 2:
// Access the first element of an array stored in memory.someArray:
memory.someArray[0]
// For memory.someArray = ["English", "Polish", "Italian"]
// the result is:
"English"
Use array methods to edit, filter, sort and get information about them.
Syntax: | Example: |
---|---|
ARRAY + . + METHOD NAME + () | memory.myArray.length() |
ARRAY
may be a literal array like this[1,2,3]
or an array stored in variable, eg.memory.someArray
METHOD NAME
is the name of one of the methods listed here.()
if there are any arguments required, they go into this brackets. If not, leave them empty.
Example:
[1, 2, "foo", "bar"].length()
// Result:
4
Full documentation on Arrays is available here
Exercise #1: Which day of the week is today?
Goal: provide the name of the current day of the week.
Quick list of steps:
- Save an array containing names of the days of the week to Memory. The names are strings, so remember about quotation marks.
- Debug by printing a chosen element using its index. Does it work as expected? This may help: Access by index.
- Use system.currentTime and getDayOfWeek() to get a current day of the week (as a number) and save it to Memory.
- Print the name of today by using the two values saved to Memory in steps 1 & 3.
- Add some nice copy to blocks and an intent “which_day” ✍️
Skills learnt
- Initializing and accessing Arrays
- Using System and DateTime API functions
Updated over 1 year ago