JavaScript
AEL (Expression language) has a lot in common with JavaScript. It uses a simplified version of JS syntax and some of its concepts, such as bracket and dot notations, one-line arrow functions, if statements, and a selection of methods to be used on strings, arrays and objects.
If you know JavaScript, you can use it instead of AEL (Expression language) while building bots in Automate.
Where you can use JS
Just like AEL, you can use JS in:
- Bot responses
- Output Conditions
- Conditional responses and Conditions in Say conditional block
- Memory values
- Commands params
- Integration input params
- and Metadata item values
How to use JS in bot responses
To enable JavaScript in bot responses, start by typing: ${ \\some code }
.
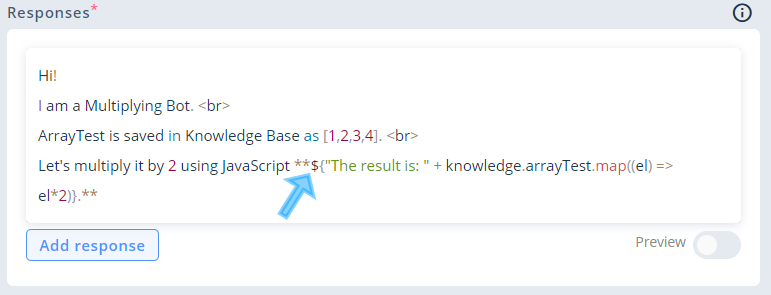
This is what this response looks like in a bot:
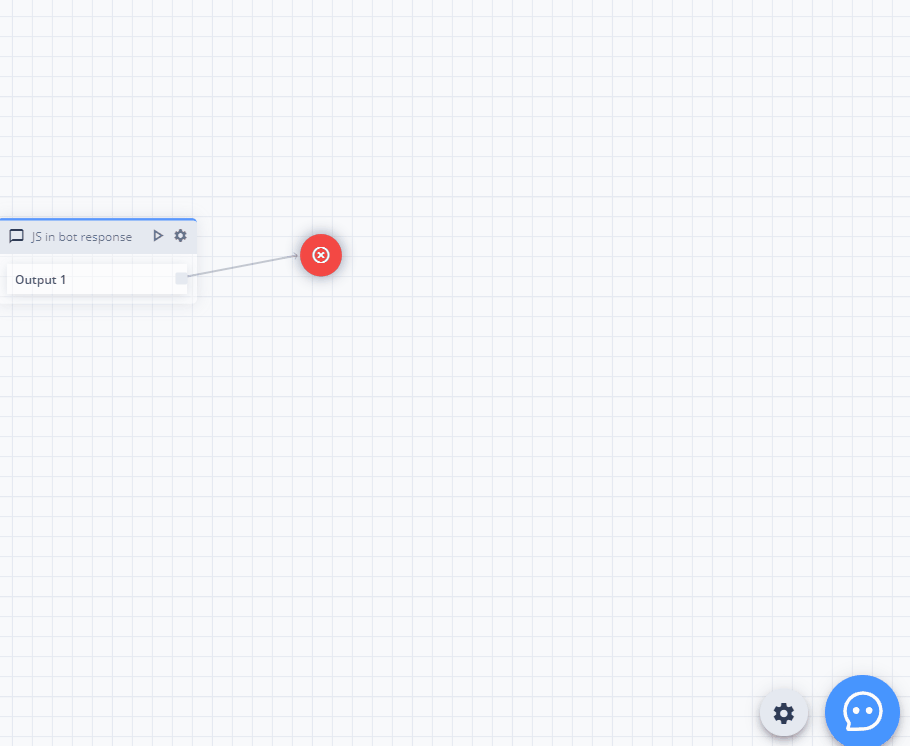
How to use JS in other fields
To enable JS in other fields use the Enable JavaScript toggle above the text editor.
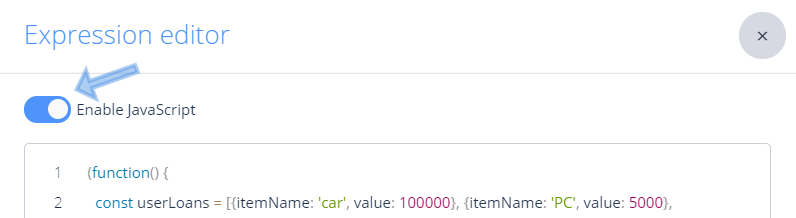
Below you can find a complete example of using JS in Output conditions:
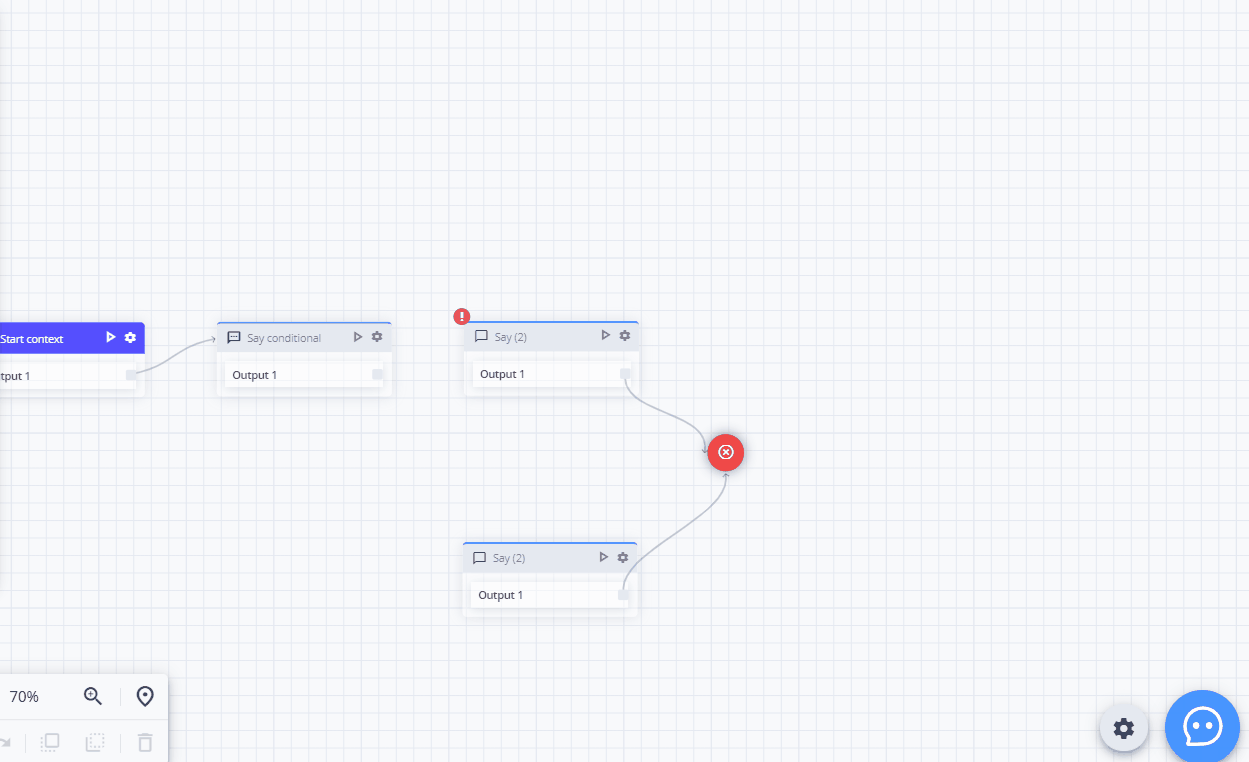
Multi-line editor
It's a joy to use JS thanks to our beautiful multi-line editor!
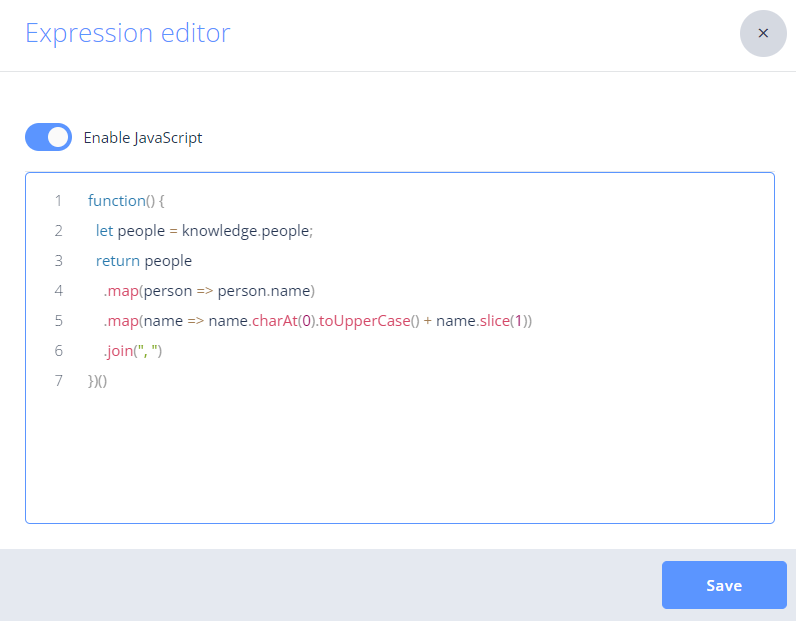
Simple expressions
If you need to write a short, one-line expression in JS, you don't need to add anything else. Here is an example of using JS to return a random number between 1 and 10:
Math.floor(Math.random() * 10)
// Result is a random number between 1 and 10
More complex code
If you wish to write more complex code that contains assignments, loops or functions, you have to put it in a function using this exact snippet:
(function() {
// put your code here
// remember to return the result
})()
(function() {
const fruitArray = [{name: "Apple", price: 10}, {name: "Grape", price: 5}, {name: "orange", price: 15}];
const moreFruitsArray = [...fruitArray, {name: "lemon", price: 2}];
const onStockFruits = moreFruitsArray.map(el => ({...el, available: true}));
const prettyPrint = (fruit) => {
return "Fruit: " + fruit.name + " price: " + fruit.price + " on stock: " +fruit.available;
};
return onStockFruits
.map(prettyPrint)
.join("\n");
})()
Fruit: Apple price: 10 on stock: true
Fruit: Grape price: 5 on stock: true
Fruit: orange price: 15 on stock: true
Fruit: lemon price: 2 on stock: true
Best practice when referencing variables from outside of JS function
Whenever you want to modify and return any more complex array or object from outside of the current JS function (eg.
memory.response
,nlu.variablesList
etc.), use spread operator to create a copy of this object to avoid problems with parsing of your JS function.(function() { let variables = [...nlu.variablesList]; return variables.filter(el => el.name = 'numeral'); })()
Possible results of a JS function
Whenever you use JS in Automate, the returned value has to have one of the AEL's data types.
โ ๏ธ The returned value of JS function cannot be:
- a function
- an object with methods as its properties
Looking for help with JS?
For a concise guide and a full reference of JavaScript, visit JavaScript Guide by MDN.
To learn JavaScript for free, visit freeCodeCamp. Be sure not to skip a section about ES6: many concepts in AEL has been taken from this version of JS.
Using AEL when you know JS
If you know JavaScript, please consider these few differences between AEL and JS:
- There are no assignment operators in AEL as it is meant to be used to write simple, one-line expressions which immediately return a value. You can't define your own functions in AEL.
- In AEL,
=
is the only equality operator, and it is not an assignment operator like in JS. - AEL is a much stronger-typed language than JS. It won't automatically convert values when JS would, except for the following two operations involving numbers:
- Comparing numbers with digits stored as a string, eg.
3 = '3'
will returntrue
. - Concatenating strings with numbers, eg.
42 + " reems of paper"
will return a string"42 reems of paper"
.
- Comparing numbers with digits stored as a string, eg.
- There is no
undefined
data type in AEL. It is replaced bynull
. If...else
statement requires theelse
part as AEL expressions always have to return a value.- Strings can only be contained within a pair of double quotation marks
""
and not single''
quotation marks.
Updated over 1 year ago
Learn basics of JavaScript and Expression language: