Objects
An Object is one of the data types available in Expression language (AEL).
Objects contain key-value pairs called "properties". A property key (name) is always a String, but the value can be of any data type. You can use Objects to store information.
You can create, access and edit Objects using AEL and JavaScript. Below, you will find all of the AEL's methods that can be used on Objects.
Properties inside an Object are not ordered, so you can't access them by their index (position), only by their keys (names).
Arrays of Objects
Arrays of Objects are a very common way to store large datasets. You can access and process them by using Array methods. Learn more about Arrays of Objects here.
Syntax
- Objects are contained within curly brackets
{}
. - Each property is a key-value pair separated by a colon
:
, like this:"someKey": true
. - Property keys (names) should be a String without any whitespaces or special characters. They can be contained within double quotation marks
""
. - Property values can be of any data type, eg. a String, a Boolean, an Array or even another Object.
- Both property keys and values can store dynamic values (eg.
system.currentTime
,nlu.intent
or variables stored in Memory). - Object properties are separated by commas
,
. Remember not to add a comma after the last property! - Spaces and line breaks are not important. An Object definition can span multiple lines - and they usually are, for better readability.
- Object's properties are unordered. It doesn't matter in which order the properties were set up.
Custom Object methods are not allowed in Automate
Normally, JavaScript allows to store functions as Object values. Such functions are called "methods".
But in Automate, methods are not allowed in both Expression language and JavaScript.
Examples
In Automate, Objects are frequently found in responses from external APIs, Knowledge Base and Memory.

Memory part 1: Creating a memory.now Object containing different formats of system.currentTime.
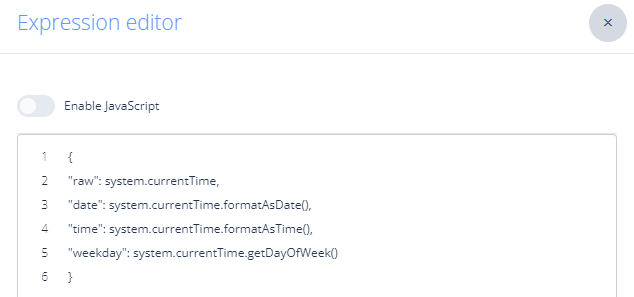
Memory part 2: Creating a memory.now
Object containing different formats of system.currentTime
.
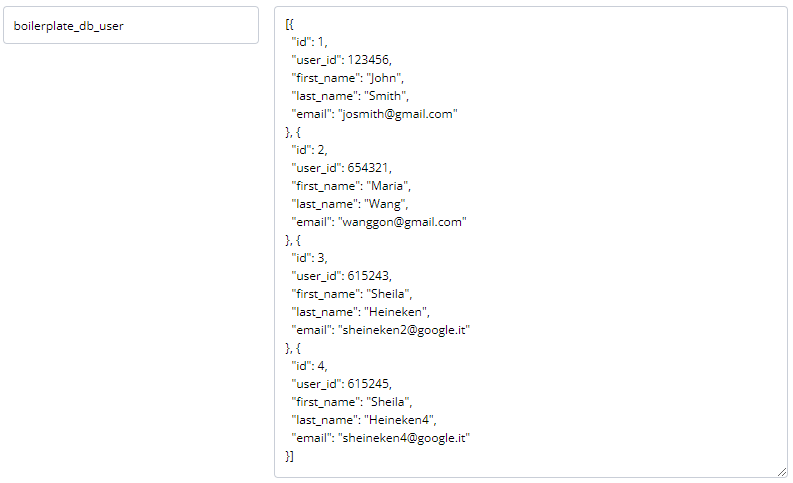
An Array of Objects stored as a Knowledge Base record.
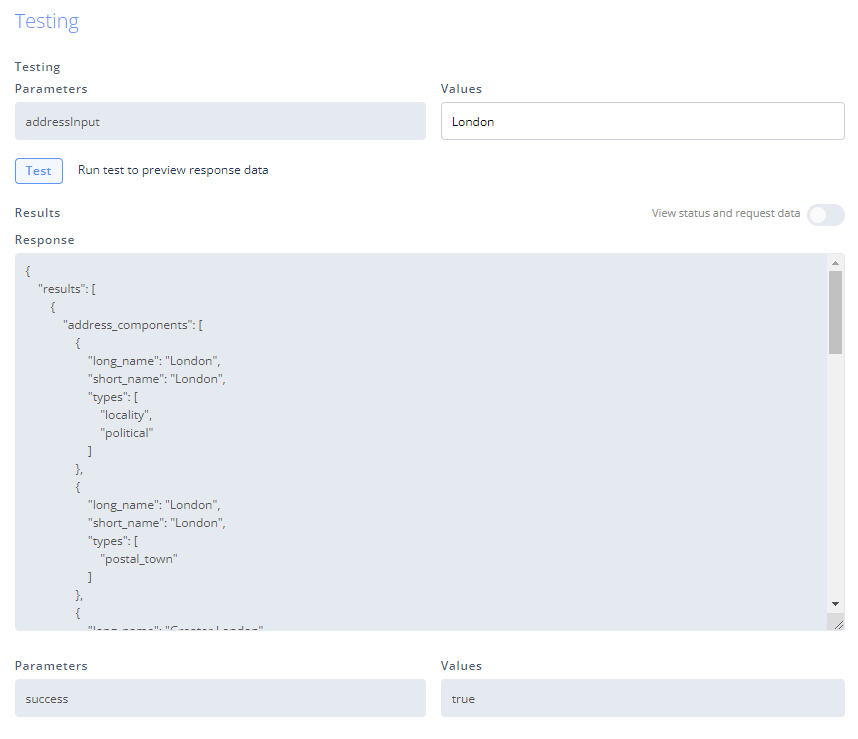
A response from Google Maps API: a JSON Object, accessible in bot's flow via memory.response
.
Full list of Object methods and operations
Method/operation | Description |
---|---|
Create an Object | Initialize an Object using literal constructor, eg. {"someKey": "someValue"}. |
Access a property | Access property value by its name using bracket or dot notation. |
Create or change a property | Add a new property to an existing Object or assign a new value to an existing property. |
property() | Access property value (including dynamic values). |
getKeys() | Get an Array with all of the Object's property names. |
Create an Object
To create an Object (e.g. when setting variable in Memory), simply input wished Object using curly brackets, colons and comas. In JS and AEL, it is called a 'literal constructor'.
Examples:
{
firstName: "Jim",
lastName: "Halpert",
age: 32,
activeEmployee: true
}
// This Object will store dynamic values from NLU API as its property values.
{
"intents": nlu.intentsList,
"variables": nlu.variablesList
}
Access a property
Access specific property value by its name using bracket or dot notation.
Dot notation
memory.someObject.propertyName
Bracket notation
memory.someObject["propertyName"]
To access a property using a dynamic value, use bracket notation without quotation marks:
memory.someObject[memory.savedPropertyName]
Properties inside an Object are not ordered, so you can't access them by their index (position), only by their keys (names).
// For an Object:
memory.employee = {
firstName: "Jim",
lastName: "Halpert",
age: 32,
activeEmployee: true
}
// Access firstName:
memory.employee.firstName
// Result:
"Jim"
// Create a String with the full name:
memory.employee.firstName + " " + memory.employee.lastName
// Result:
"Jim Halpert"
// For an Object:
memory.employee = {
firstName: "Jim",
lastName: "Halpert",
age: 32,
activeEmployee: true
}
// and for an intent recognized by NLU:
nlu.intent = "age"
// Access a property defined by recognized intent:
memory.employee[nlu.intent]
// Result:
32
Create or change a property
Create a property or update a value of an existing property of an Object stored in Memory using dot notation. As a key, input an Object's name followed by a dot and a property's name to be created or updated.
Example
After being created in the 1st input, the Objectmemory.employee1
contains 4 properties. The 2nd input adds a 5th property yearlyBonus
and sets its value to $240
. The 3rd input updates age
by adding 1 to its previous value.
Results of printing {memory.employee1}
in a response after each input:
{
firstName: "Jim",
lastName: "Halpert",
age: 32,
activeEmployee: true
}
{
firstName: "Jim",
lastName: "Halpert",
age: 32,
activeEmployee: true,
yearlyBonus: "$240"
}
{
firstName: "Jim",
lastName: "Halpert",
age: 33,
activeEmployee: true,
yearlyBonus: "$240"
}
Manipulating object with dynamic keys
In more advanced cases it may happen that you will need to manipulate object with dynamic keys. In such case use JavaScript to assign value.
(function() {
let params = {};
for (const [key, value] of Object.entries(memory.parameters)) {
params[key] = value
}
params[memory.index.toString()] = system.userInput;
return params;
})()
Above example shows use case where in a loop user is asked to provide values that are stored in memory.parameters
for each iteration. As a result you receive object like this:
{
"1" : "https://sentione.com",
"0" : "6"
}
property()
If property name is dynamic (e.g. other memory field and/or it's not valid name) then we can use method property()
Access specific property value by its name given as an argument (inside the brackets). This method accepts dynamic values (eg. other memory values or NLU results).
// For an Object:
memory.employee = {
firstName: "Jim",
lastName: "Halpert",
age: 32,
activeEmployee: true
}
// and for an intent recognized by NLU:
nlu.intent = "age"
// Access a property defined by recognized intent:
memory.employee.property(nlu.intent)
// Result:
32
getKeys()
Create an Array containing all of the Object's property keys.
As Object's properties are always unordered, elements in the created Array may have different order than that in which the user defined them in an Object or how they look like when the Object is printed in a response.
// For an Object:
memory.employee = {
firstName: "Jim",
lastName: "Halpert",
age: 32,
activeEmployee: true
}
// Create an Array using getKeys():
memory.employee.getKeys();
// Result:
[
"activeEmployee",
"firstName",
"age",
"lastName"
]
Updated 5 months ago
Learn more about Expression language and its methods & APIs: